first: 通过以下代码读入外部词云形状图片(需要先pip3 install
imageio安装imageio)
1 2 3
| import imageio mk=imageio.imread("picture.png") w=wordcloud.WordCloud(mask=mk)
|
例子
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| import jieba import wordcloud import imageio
mk=imageio.imread("/Users/SJCHEN/Downloads/zihaowordcloud-master/code/wujiaoxing.png") w=wordcloud.WordCloud(mask=mk)
w=wordcloud.WordCloud(width=1000, height=700, background_color='white', font_path='Songti.ttc', mask=mk, scale=15) f=open('/Users/SJCHEN/Downloads/zihaowordcloud-master/code/关于实施乡村振兴战略的意见.txt',encoding='utf-8') txt=f.read() textlist=jieba.lcut(txt) string=" ".join(textlist)
w.generate(string) w.to_file('example7.png')
|
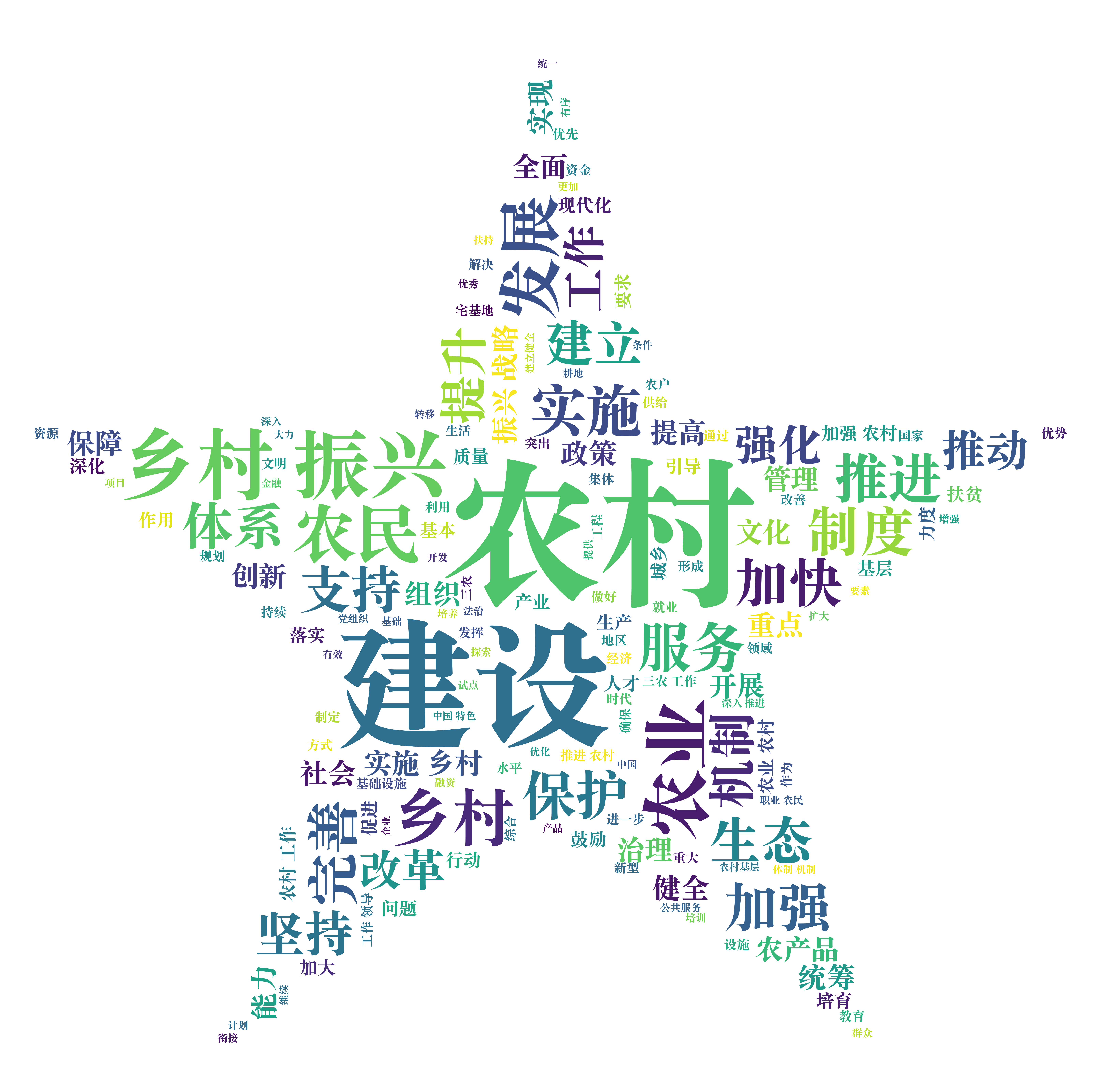
去除指定词(stopwords参数去除词)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| import jieba import wordcloud import imageio
mk=imageio.imread("/Users/SJCHEN/Downloads/zihaowordcloud-master/code/chinamap.png") w=wordcloud.WordCloud(mask=mk)
w=wordcloud.WordCloud(width=1000, height=700, background_color='white', font_path='Songti.ttc', mask=mk, scale=15, stopwords={'曹操','孔明'}) f=open('/Users/SJCHEN/Downloads/zihaowordcloud-master/code/三国演义.txt',encoding='utf-8') txt=f.read() textlist=jieba.lcut(txt) string=" ".join(textlist)
w.generate(string) w.to_file('example10.png')
|
利用原来的方法我们得到
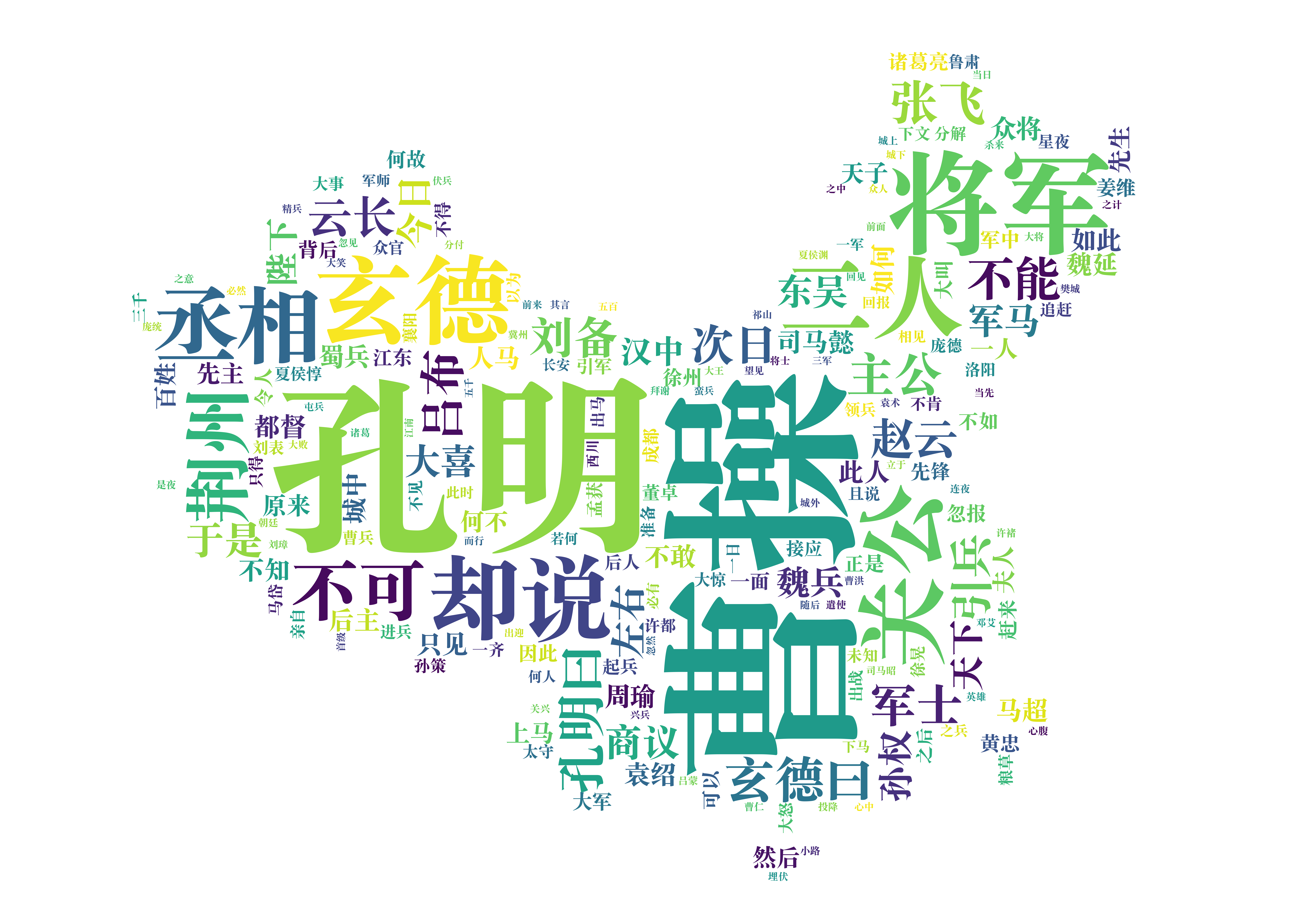
去掉指定的词后

勾勒轮廓线
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| import wordcloud import imageio
mk=imageio.imread("/Users/SJCHEN/Downloads/zihaowordcloud-master/code/alice.png")
w=wordcloud.WordCloud(background_color='white', mask=mk, contour_width=1, contour_color='steelblue')
string =open('/Users/SJCHEN/Downloads/zihaowordcloud-master/code/hamlet.txt').read() w.generate(string) w.to_file('example11.png')
|
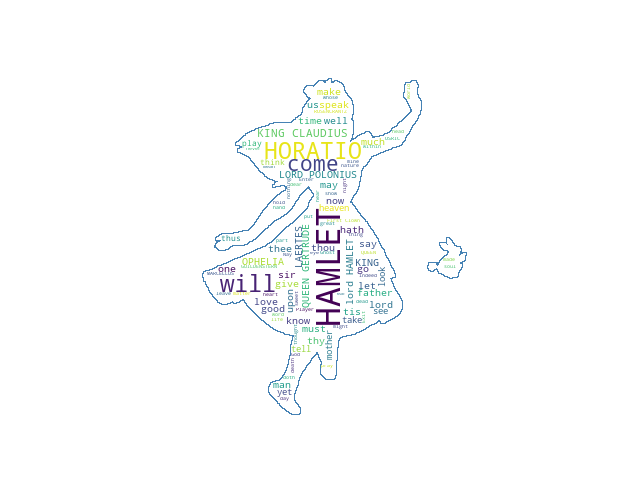
按模版填色
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35
| import matplotlib.pyplot as plt from wordcloud import WordCloud,ImageColorGenerator import imageio
text = open('/Users/SJCHEN/Downloads/zihaowordcloud-master/code/alice.txt',encoding='utf-8').read()
mk = imageio.imread("/Users/SJCHEN/Downloads/zihaowordcloud-master/code/alice_color.png")
wc = WordCloud(background_color="white", mask=mk,)
wc.generate(text)
image_colors = ImageColorGenerator(mk)
fig, axes = plt.subplots(1, 3)
axes[0].imshow(wc)
axes[1].imshow(wc.recolor(color_func=image_colors), interpolation="bilinear")
axes[2].imshow(mk, cmap=plt.cm.gray) for ax in axes: ax.set_axis_off() plt.show()
wc_color = wc.recolor(color_func=image_colors)
wc_color.to_file('example11.png')
|

如果只是简单的用的话
1 2 3 4 5 6 7 8 9 10 11
| from wordcloud import WordCloud,ImageColorGenerator import imageio
text = open('/Users/SJCHEN/Downloads/zihaowordcloud-master/code/alice.txt',encoding='utf-8').read() mk=imageio.imread("/Users/SJCHEN/Downloads/zihaowordcloud-master/code/alice_color.png") w=WordCloud(background_color='white', mask=mk,) w.generate(text) image_colors=ImageColorGenerator(mk) w_color=w.recolor(color_func=image_colors) w_color.to_file('exam.png')
|